What's new in Xcode 13 beta 4
Xcode 13 beta 4 has just been released: let's have a look at what's new in SwiftUI!
Bordered Button
style updates
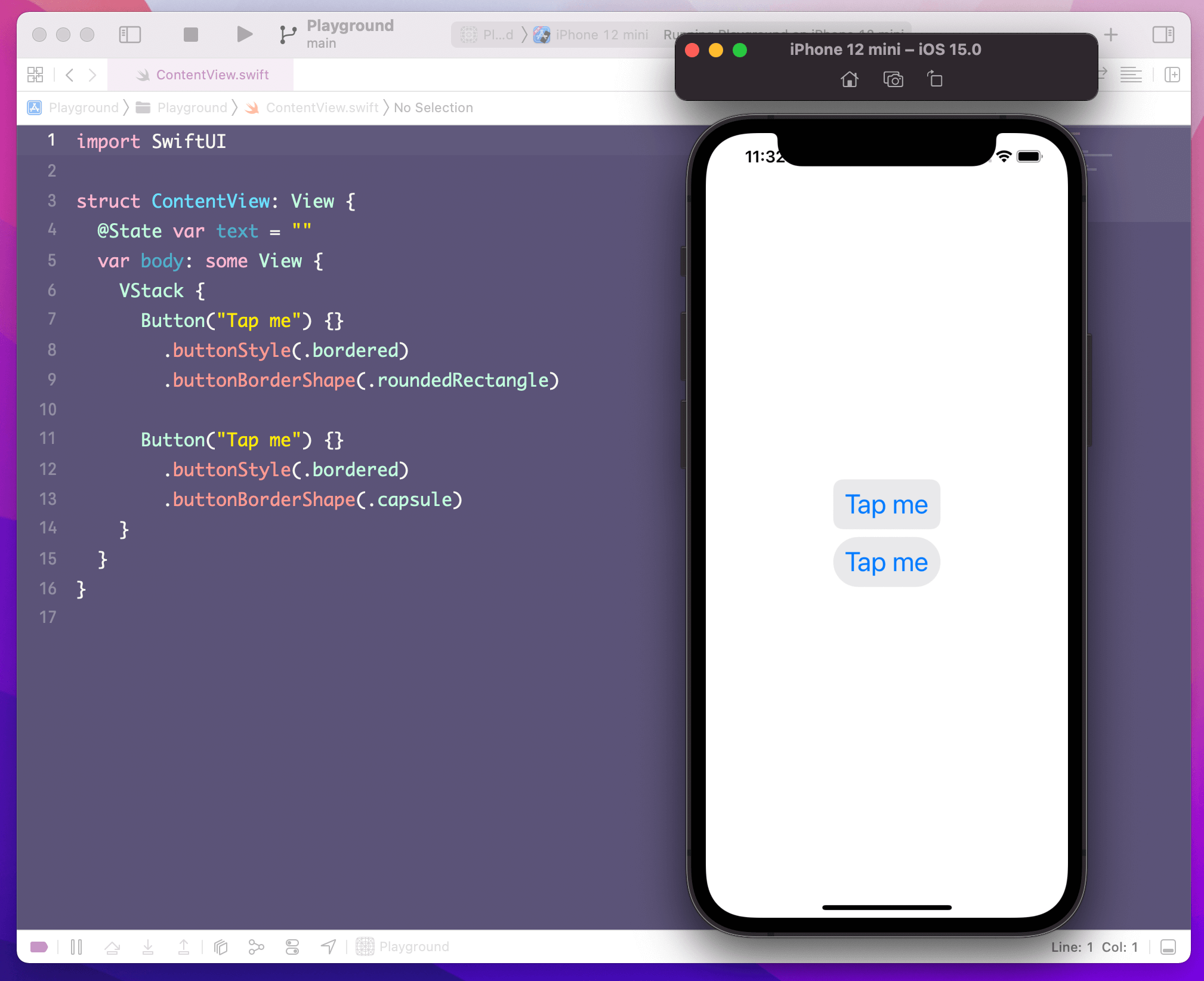
A new buttonBorderShape(_:)
view modifier has been introduced, replacing the previous BorderedButtonStyle
shape
parameter:
From:
Button("Tap me") {}
.buttonStyle(BorderedButtonStyle(shape: .roundedRectangle))
Button("Tap me") {}
.buttonStyle(BorderedButtonStyle(shape: .capsule))
To:
Button("Tap me") {}
.buttonStyle(.bordered)
.buttonBorderShape(.roundedRectangle)
Button("Tap me") {}
.buttonStyle(.bordered)
.buttonBorderShape(.capsule)
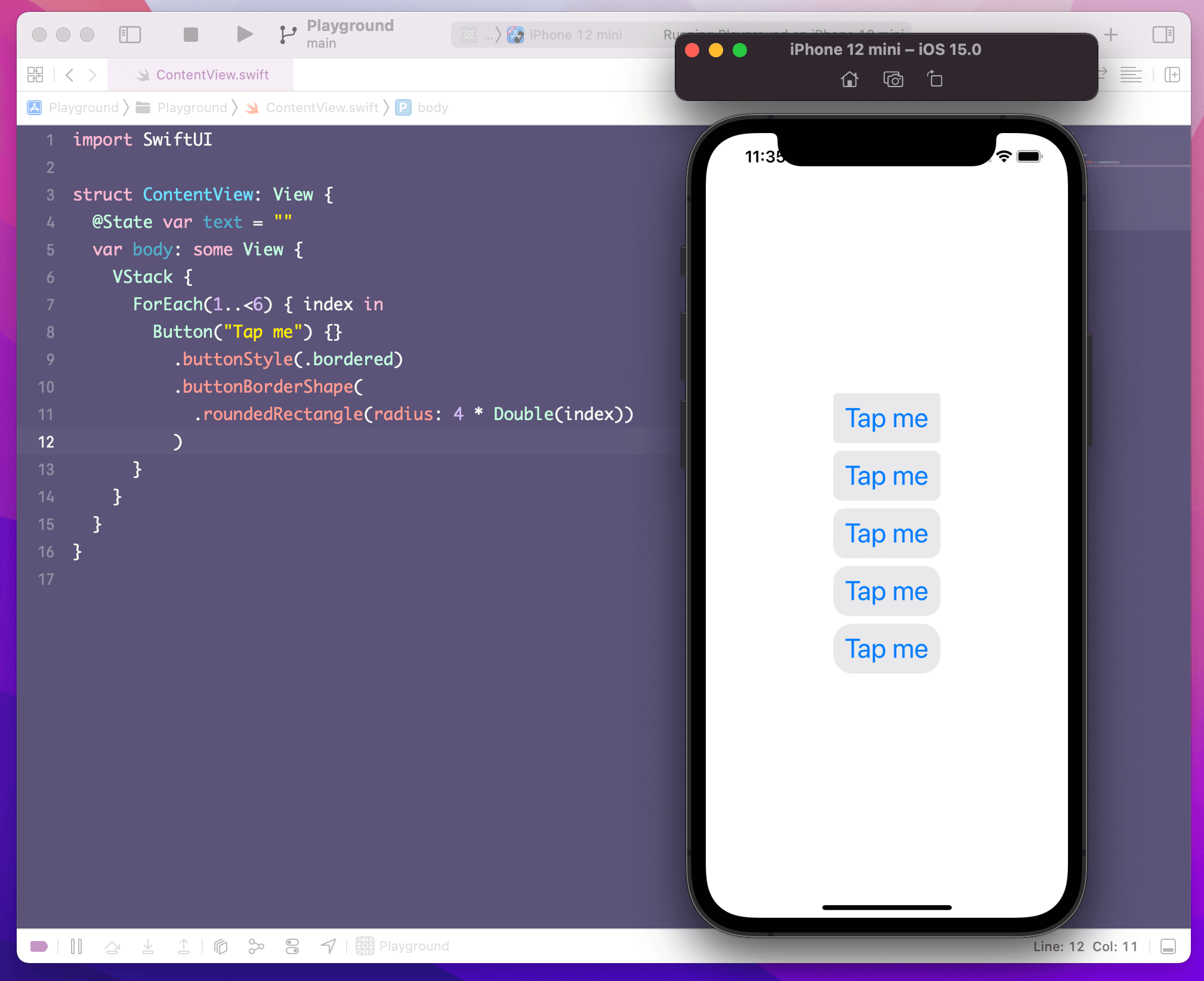
The new buttonBorderShape(_:)
parameter type, ButtonBorderShape
, comes with a new roundedRectangle(radius: CGFloat)
option, meaning that we can now customize the button radius:
ForEach(1..<6) { index in
Button("Tap me") {}
.buttonStyle(.bordered)
.buttonBorderShape(.roundedRectangle(radius: 4 * Double(index)))
}
As of Xcode 13b4, there's no public API to read the environment
BorderedButtonStyle
value, FB9413086.
Control prominence updates
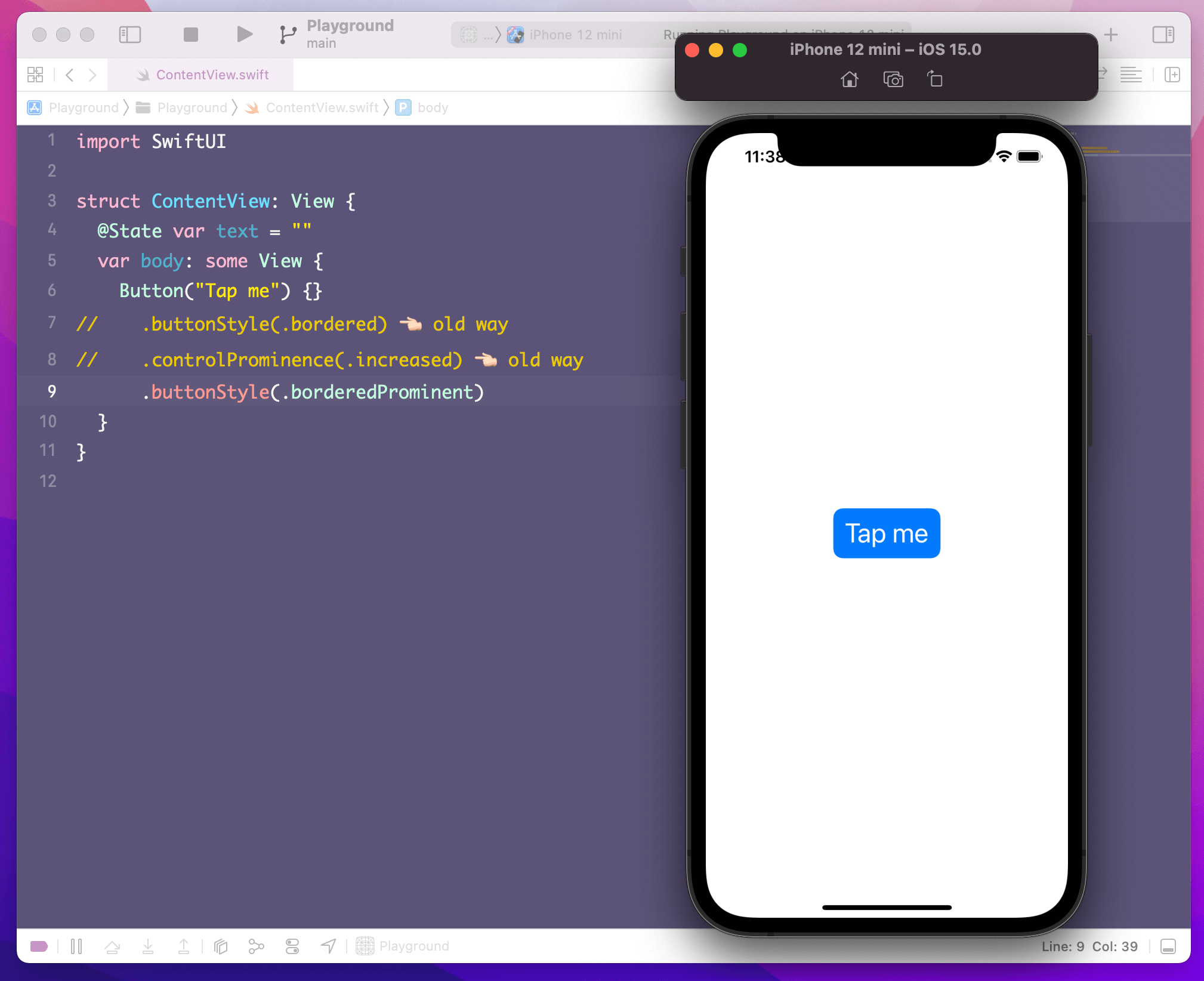
The controlProminence(_:)
modifier has been deprecated in favor of a new .borderedProminent
button style.
From:
Button("Tap me") {}
.buttonStyle(.bordered)
.controlProminence(.increased)
To:
Button("Tap me") {}
.buttonStyle(.borderedProminent)
Prominence
is still used used with for (List
's) headerProminence(_:)
modifier.
controlProminence
environment value has not yet been deprecated, FB9413125.
New strikethrough and underline AttributedString
styles
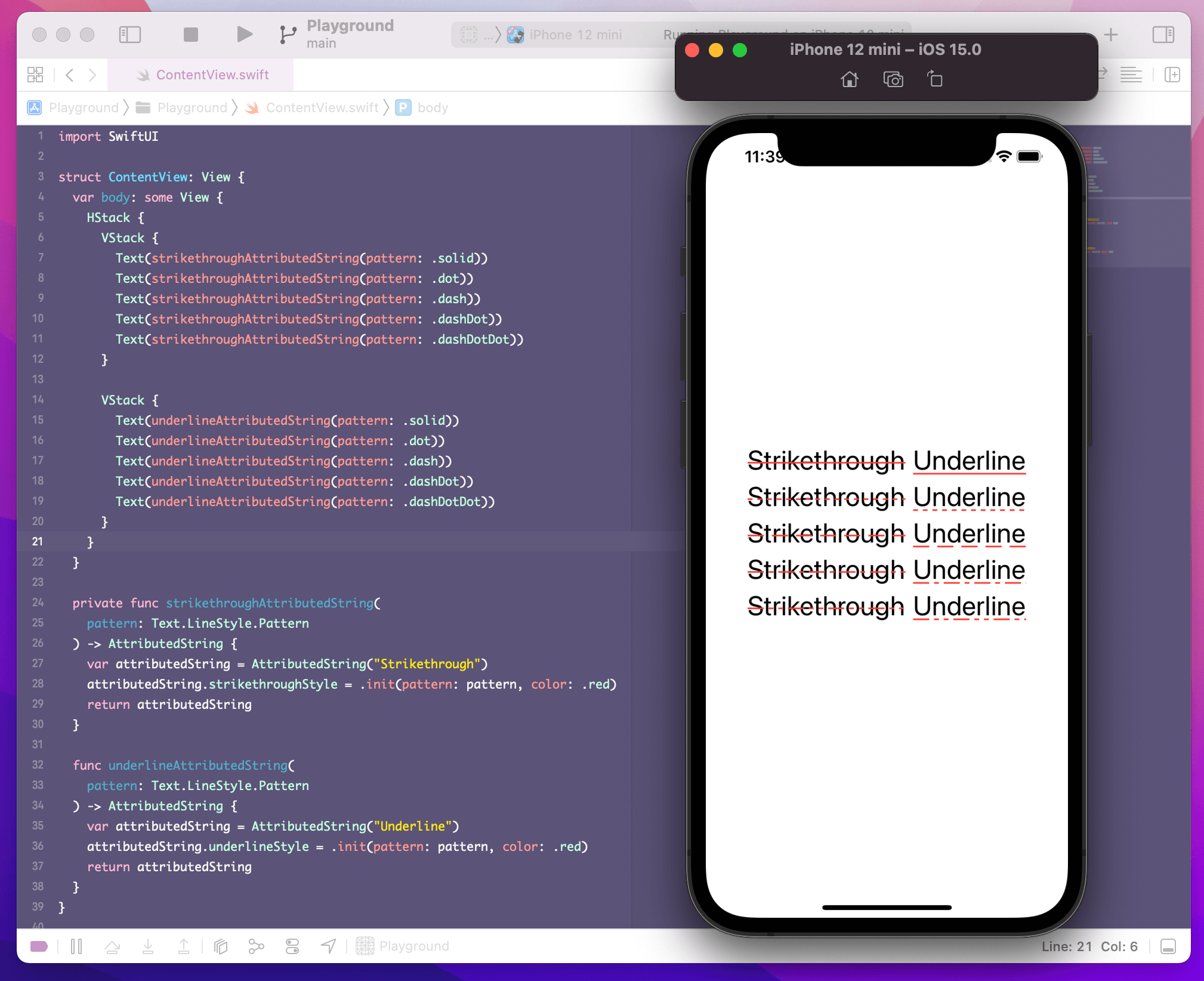
AttributeString
s can now further customize the strikethrough and underline lines with a new Text.LineStyle.Pattern
type, here are all the new styles:
struct ContentView: View {
var body: some View {
HStack {
VStack {
Text(strikethroughAttributedString(pattern: .solid))
Text(strikethroughAttributedString(pattern: .dot))
Text(strikethroughAttributedString(pattern: .dash))
Text(strikethroughAttributedString(pattern: .dashDot))
Text(strikethroughAttributedString(pattern: .dashDotDot))
}
VStack {
Text(underlineAttributedString(pattern: .solid))
Text(underlineAttributedString(pattern: .dot))
Text(underlineAttributedString(pattern: .dash))
Text(underlineAttributedString(pattern: .dashDot))
Text(underlineAttributedString(pattern: .dashDotDot))
}
}
}
private func strikethroughAttributedString(
pattern: Text.LineStyle.Pattern
) -> AttributedString {
var attributedString = AttributedString("Strikethrough")
attributedString.strikethroughStyle = .init(pattern: pattern, color: .red)
return attributedString
}
func underlineAttributedString(
pattern: Text.LineStyle.Pattern
) -> AttributedString {
var attributedString = AttributedString("Underline")
attributedString.underlineStyle = .init(pattern: pattern, color: .red)
return attributedString
}
}
With this update,
AttributedString
'sstrikethroughColor
andunderlineColor
have been deprecated.
Text field auto capitalization gains a full-SwiftUI modifier
SwiftUI's autocapitalization(_:)
is now deprecated in favor of a new textInputAutocapitalization(_:)
modifier.
From:
TextField("All characters auto capitalization", text: $text)
.autocapitalization(.allCharacters)
To:
TextField("All characters auto capitalization", text: $text)
.textInputAutocapitalization(.characters)
The new modifier uses a new TextInputAutocapitalization
SwiftUI struct:
struct TextInputAutocapitalization {
/// Defines an autocapitalizing behavior that will not capitalize anything.
public static var never: TextInputAutocapitalization { get }
/// Defines an autocapitalizing behavior that will capitalize the first
/// letter of every word.
public static var words: TextInputAutocapitalization { get }
/// Defines an autocapitalizing behavior that will capitalize the first
/// letter in every sentence.
public static var sentences: TextInputAutocapitalization { get }
/// Defines an autocapitalizing behavior that will capitalize every letter.
public static var characters: TextInputAutocapitalization { get }
}
While the deprecated modifier used UIKit's UITextAutocapitalizationType
:
public enum UITextAutocapitalizationType : Int {
case none = 0
case words = 1
case sentences = 2
case allCharacters = 3
}
Documentation
We have a lot more documentation for:
Task
view modifiersAccessibilityChildBehavior
properties (combine
,ignore
,contain
)
Conclusions
Did you find anything else interesting in Xcode 13 beta 4? Please let me know!
As always, please report any issue to Apple: we're still months away from the official release, things can and will be fixed, as long as we report them.
This article is part of a series exploring new SwiftUI features. We will cover many more during the rest of the summer: subscribe to Five Stars's feed RSS or follow @FiveStarsBlog on Twitter to never miss new content!