What's new in Xcode 13 beta 2
Xcode 13 beta 2 has just been released, and it comes with new SwiftUI goodies: let's have a look at what's new!
textSelection
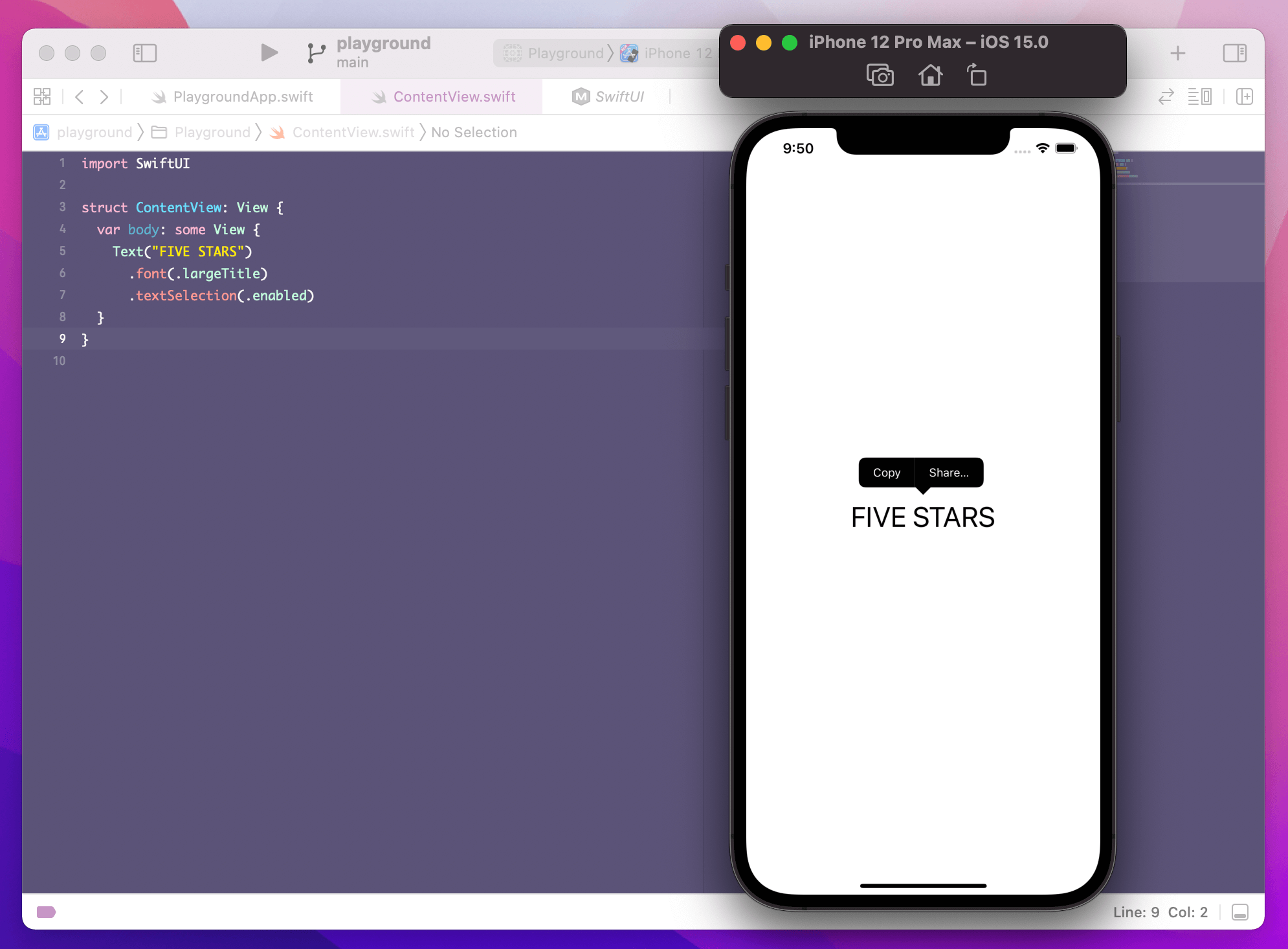
struct ContentView: View {
var body: some View {
Text("FIVE STARS")
.font(.largeTitle)
.textSelection(.enabled)
}
}
The textSelection(_:)
modifier is now available on iOS, this view modifier allows users to select/copy the content of Text
views.
Mini ControlSize
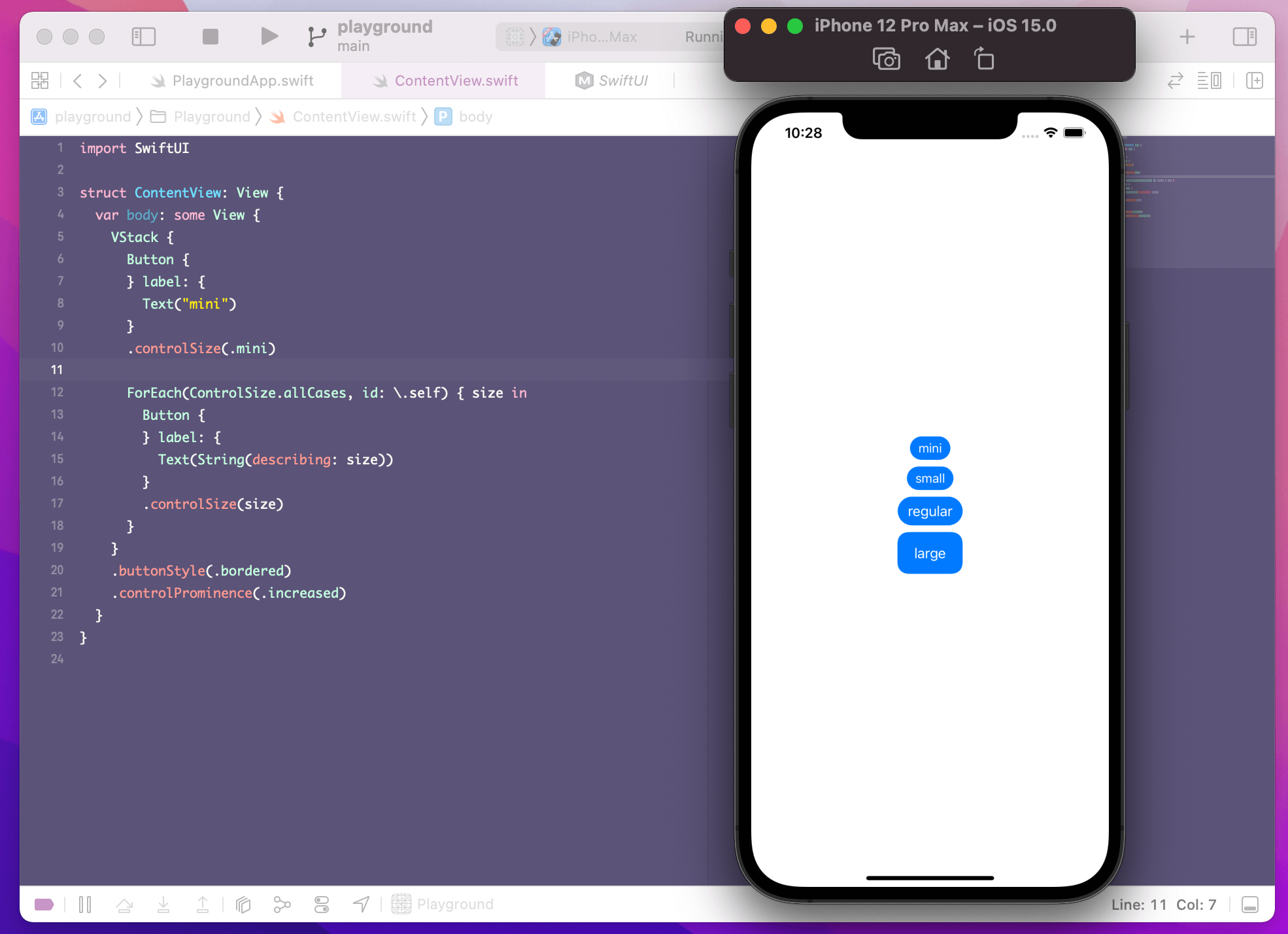
struct ContentView: View {
var body: some View {
VStack {
ForEach(ControlSize.allCases, id: \.self) { size in
Button {
} label: {
Text(String(describing: size))
}
.controlSize(size)
.controlSize(size)
}
}
.buttonStyle(.bordered)
.controlProminence(.increased)
}
}
We have a new .mini
ControlSize
:
it doesn't seem to be different than the .small
size in this specific example, but it might in other systems/contexts.
As of Xcode 13b2,
.mini
is not part ofControlSize.allCases
(FB9208000). Update: Xcode 13b3 fixes this issue.
dismissSearch
struct ContentView: View {
@State private var query = ""
@Environment(\.dismissSearch) private var dismissSearch
var body: some View {
NavigationView {
List {
// ...
}
.searchable(text: $query) {
}
.toolbar {
Button("Cancel") {
dismissSearch()
}
}
}
}
}
There's a new dismissSearch
environment value which, when triggered:
- will set
isSearching
tofalse
- any search text will be cleared
- any search field will lose focus
presentationMode deprecation
extension EnvironmentValues {
@available(iOS, introduced: 13.0, deprecated: 100000.0, message: "Use isPresented or dismiss")
@available(macOS, introduced: 10.15, deprecated: 100000.0, message: "Use isPresented or dismiss")
@available(tvOS, introduced: 13.0, deprecated: 100000.0, message: "Use isPresented or dismiss")
@available(watchOS, introduced: 6.0, deprecated: 100000.0, message: "Use isPresented or dismiss")
public var presentationMode: Binding<PresentationMode> { get }
}
presentationMode
has been soft deprecated in favor of the new isPresented
and dismiss
environment values.
This is something I suggested/mentioned in What's new in SwiftUI.
Text format initializer
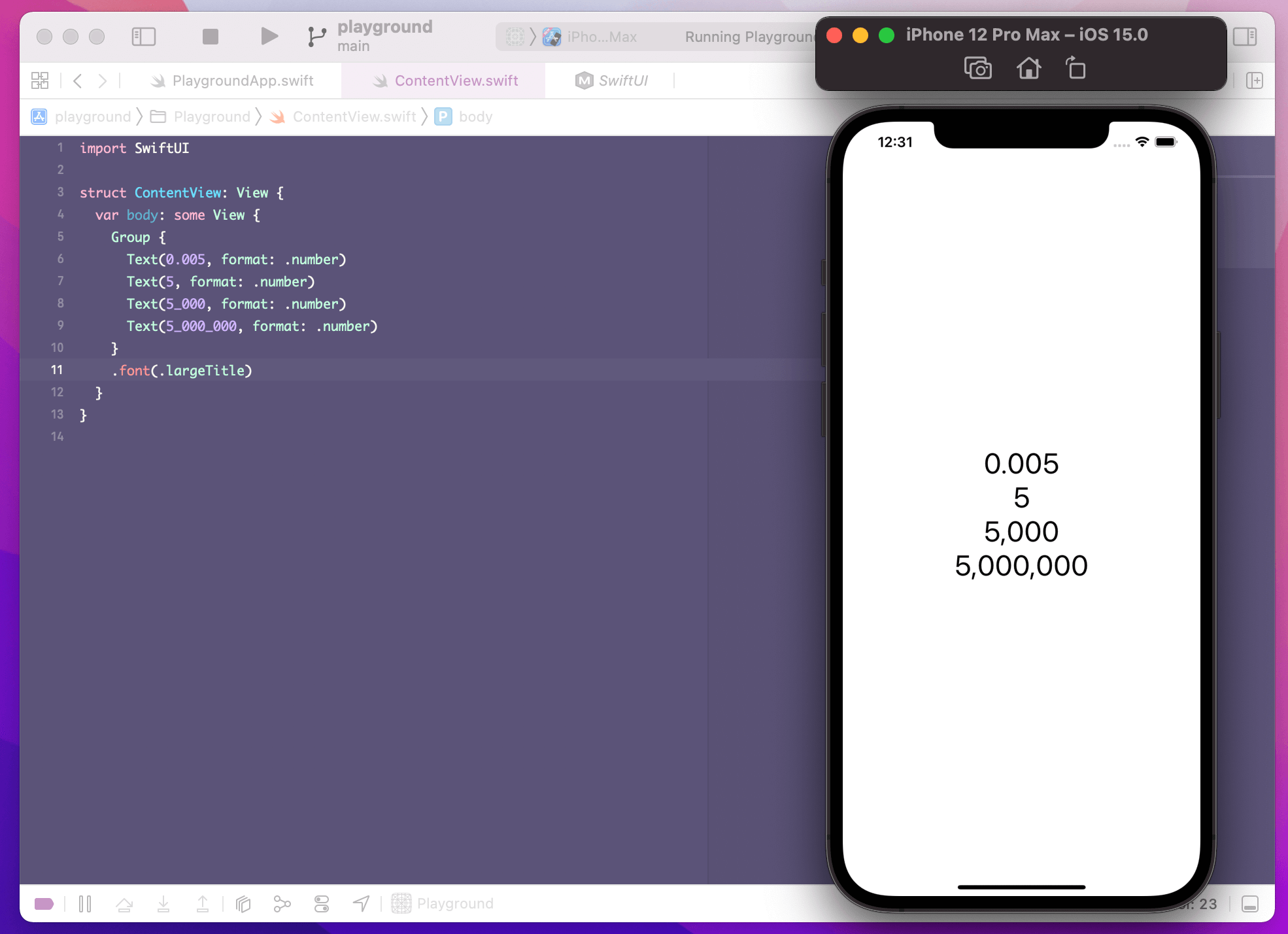
struct ContentView: View {
var body: some View {
Text(0.005, format: .number)
Text(5, format: .number)
Text(5_000, format: .number)
Text(5_000_000, format: .number)
}
}
Text
has gained a new init(_:format:)
initializer, specifically dedicated to formatting values.
Searchable Prompt
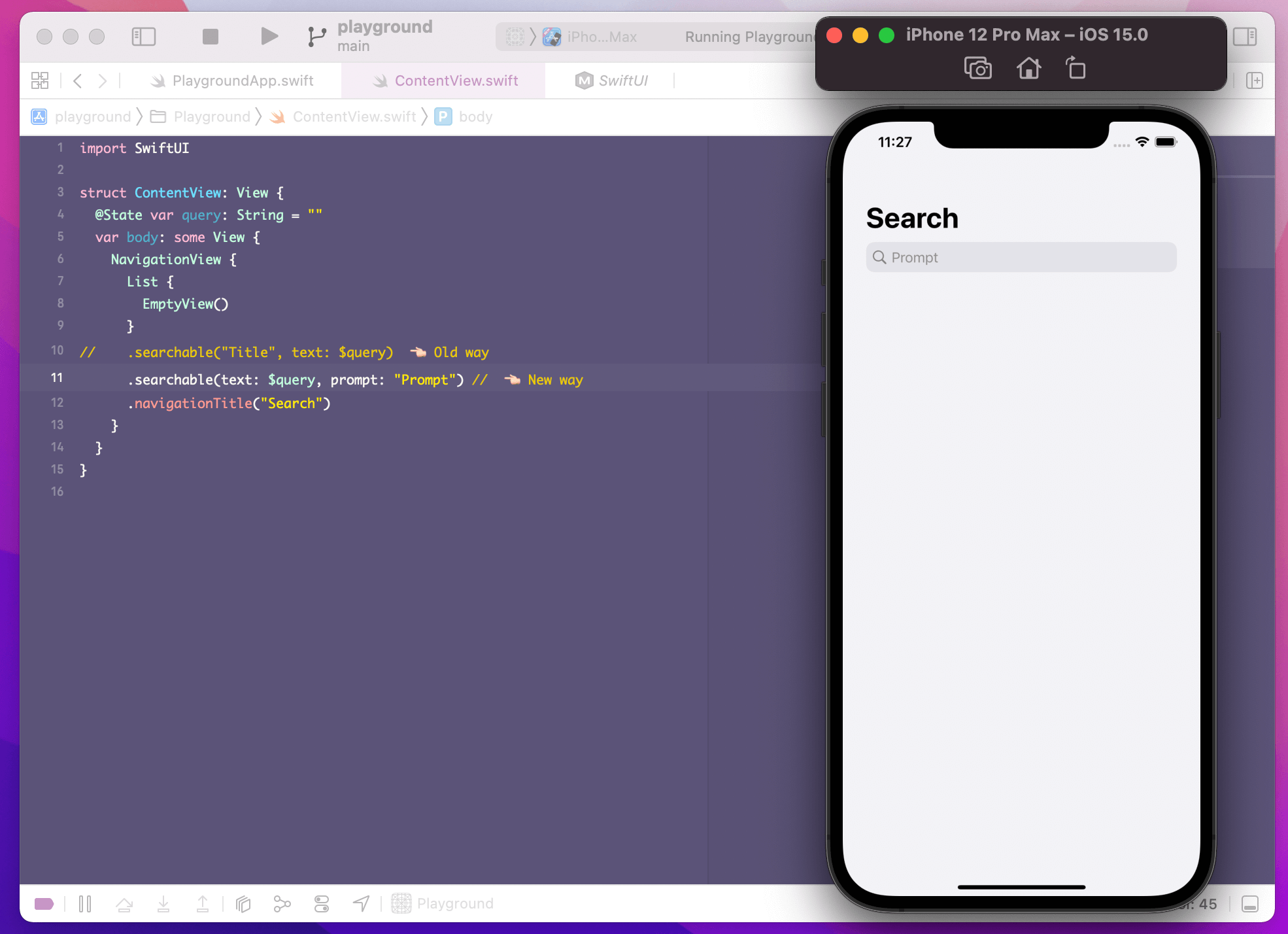
struct ContentView: View {
@State var query: String = ""
var body: some View {
NavigationView {
List {
EmptyView()
}
// .searchable("Title", text: $query) 👈🏻 Old way
.searchable(text: $query, prompt: "Prompt") // 👈🏻 New way
.navigationTitle("Search")
}
}
}
Similar to TextField
s, also the searchable
modifier is now embracing prompts instead of titles.
Conclusions
iOS 15 is still a few months away: while we might get even more features in upcoming betas, this is the perfect time to test out our apps and report any beta issue we spot to Apple.
Did you find anything else interesting in Xcode 13 beta 2? Please let me know!
This article is part of a series exploring new SwiftUI features. We will cover many more during the rest of the summer: subscribe to Five Stars's feed RSS or follow @FiveStarsBlog on Twitter to never miss new content!